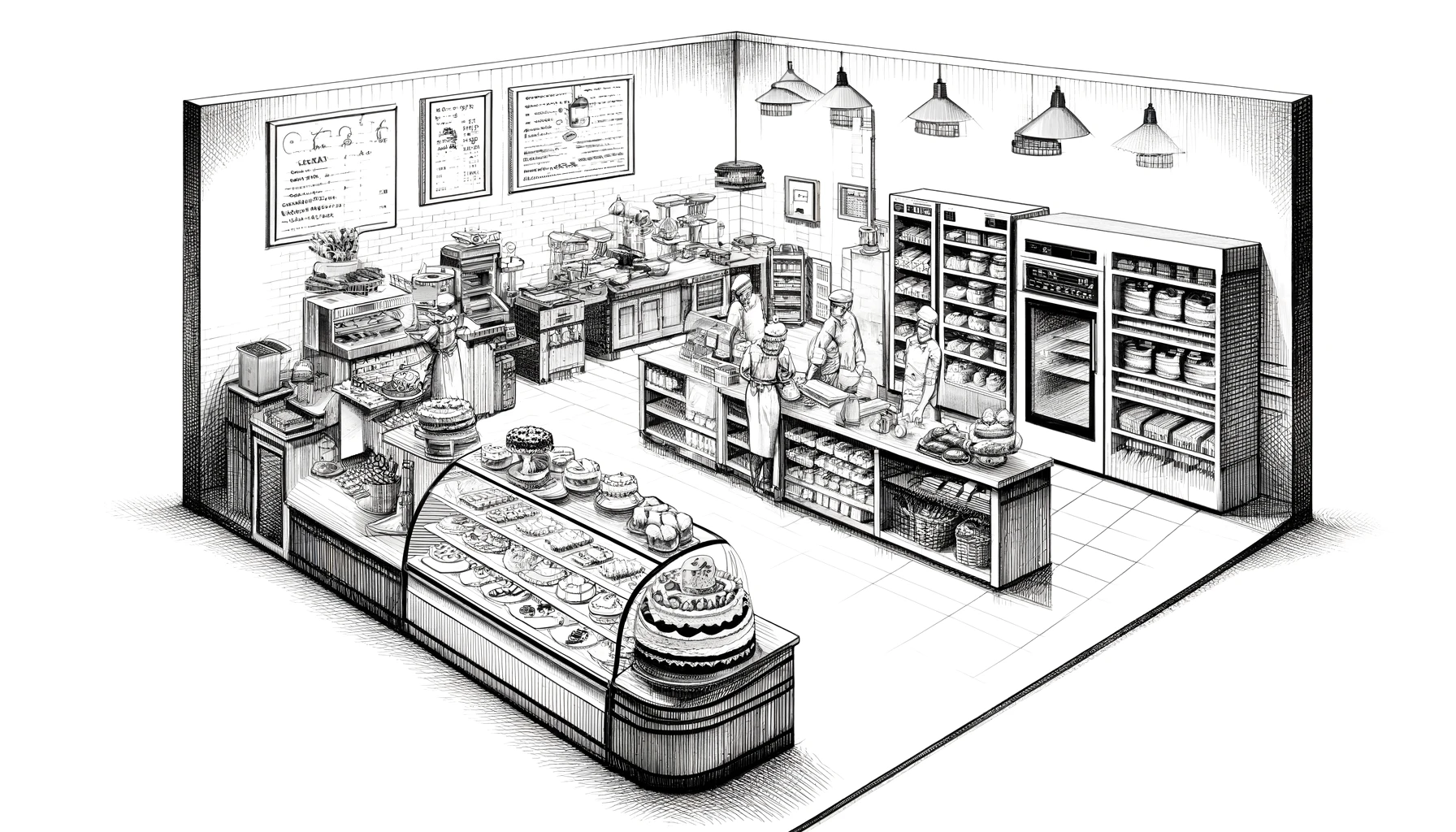
Understanding Lexical Environments in JavaScript: A Bakery Analogy
Imagine a bustling bakery where each section, from the front counter to the kitchen, has its own specific responsibilities and ingredients. This bakery will help us understand the concept of lexical environments in JavaScript, a crucial aspect of how JavaScript manages variables and their scopes.
The Bakery Layout
- Front Counter (Global Scope): This is where customers place their orders and pick up their baked goods. It's the most accessible area, and everyone in the bakery can see what’s happening here.
- Bakery Kitchen (Function Scope): This is where the magic happens. Bakers mix ingredients and bake the goods. It's a restricted area, only accessible to those working in the kitchen.
- Storage Room (Block Scope): This is where ingredients and supplies are stored. It's even more restricted, usually only accessible to specific staff members like the head baker.
Lexical Environments in JavaScript
In JavaScript, a lexical environment is like each section of our bakery. It’s an area where variables (ingredients) are stored and managed. Let's break down this concept using our bakery analogy.
Global Scope: Front Counter
1let bakeryName = "Sweet Treats Bakery";
2
3function showBakeryName() {
4 console.log(bakeryName);
5}
The bakeryName variable is declared at the front counter (global scope). Everyone in the bakery, including the bakers in the kitchen and the staff in the storage room, can see and use bakeryName.
Function Scope: Bakery Kitchen
1function bakeCake() {
2 let ingredients = "flour, sugar, eggs";
3 console.log(`Baking a cake with ${ingredients}`);
4}
Inside the bakeCake function, we have the ingredients variable, which is only accessible in the bakery kitchen (function scope). No one outside the kitchen can directly use ingredients. This isolation ensures that the kitchen can work efficiently without interference.
Block Scope: Storage Room
1function bakePastry() {
2 let tools = "rolling pin, mixer";
3
4 if (true) {
5 let secretIngredient = "cinnamon";
6 console.log(`Adding ${secretIngredient} to the pastry`);
7 }
8
9 // console.log(secretIngredient); // Error: secretIngredient is not defined
10}
Within the bakePastry function, we declare tools which is accessible within the entire kitchen (function scope). However, secretIngredient is stored in a storage room (block scope) within an if block. Only those who have access to this specific storage room can use secretIngredient.
How Lexical Environments Work
- Environment Records: Each section of our bakery keeps a record of its ingredients (variables). For example, the kitchen keeps track of ingredients, and the storage room keeps track of secretIngredient.
- Outer Environment Reference: Each section has a reference to the front counter (global scope). If a baker needs to know the bakery name, they can refer to the global scope. However, the front counter doesn't have access to what's happening inside the kitchen or the storage room unless explicitly shared.
Here&pos;s an example:
1let bakeryName = "Sweet Treats Bakery";
2
3function bakeCake() {
4 let ingredients = "flour, sugar, eggs";
5
6 function mixIngredients() {
7 let tool = "mixer";
8 console.log(`Mixing ${ingredients} with a ${tool} at ${bakeryName}`);
9 }
10
11 mixIngredients();
12 // console.log(tool); // Error: tool is not defined
13}
14
15bakeCake();
In this example:
- The global scope (bakeryName) is like the front counter.
- The bakeCake function scope is like the kitchen, holding ingredients.
- The mixIngredients function has its own scope (like a specific workstation within the kitchen) with tool.
The mixIngredients function can access ingredients from its outer environment (the kitchen) and bakeryName from the global scope (the front counter). However, the kitchen cannot access tool directly.
Summary
Understanding lexical environments in JavaScript through our bakery analogy helps demystify how variable scopes work. Each section of the bakery has its own set of variables, with specific rules about what can be accessed and from where. This structure ensures that variables are managed efficiently and securely, just like ingredients in a well-organized bakery.
Happy coding!